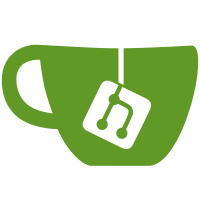
The EngineCache class replaces all previously individual solutions for caches in the context of the engines. - demo_offline.py - duckduckgo.py - radio_browser.py - soundcloud.py - startpage.py - wolframalpha_api.py - wolframalpha_noapi.py Search term to test most of the modified engines:: !ddg !rb !sc !sp !wa test !ddg !rb !sc !sp !wa foo For introspection of the DB, jump into developer environment and run command to show cache state:: $ ./manage pyenv.cmd bash --norc --noprofile (py3) python -m searx.enginelib cache state cache tables and key/values =========================== [demo_offline ] 2025-04-22 11:32:50 count --> (int) 4 [startpage ] 2025-04-22 12:32:30 SC_CODE --> (str) fSOBnhEMlDfE20 [duckduckgo ] 2025-04-22 12:32:31 4dff493e.... --> (str) 4-128634958369380006627592672385352473325 [duckduckgo ] 2025-04-22 12:40:06 3e2583e2.... --> (str) 4-263126175288871260472289814259666848451 [radio_browser ] 2025-04-23 11:33:08 servers --> (list) ['https://de2.api.radio-browser.info', ...] [soundcloud ] 2025-04-29 11:40:06 guest_client_id --> (str) EjkRJG0BLNEZquRiPZYdNtJdyGtTuHdp [wolframalpha ] 2025-04-22 12:40:06 code --> (str) 5aa79f86205ad26188e0e26e28fb7ae7 number of tables: 6 number of key/value pairs: 7 In the "cache tables and key/values" section, the table name (engine name) is at first position on the second there is the calculated expire date and on the third and fourth position the key/value is shown. About duckduckgo: The *vqd coode* of ddg depends on the query term and therefore the key is a hash value of the query term (to not to store the raw query term). In the "properties of ENGINES_CACHE" section all properties of the SQLiteAppl / ExpireCache and their last modification date are shown:: properties of ENGINES_CACHE =========================== [last modified: 2025-04-22 11:32:27] DB_SCHEMA : 1 [last modified: 2025-04-22 11:32:27] LAST_MAINTENANCE : [last modified: 2025-04-22 11:32:27] crypt_hash : ca612e3566fdfd7cf7efe2b1c9349f461158d07cb78a3750e5c5be686aa8ebdc [last modified: 2025-04-22 11:32:30] CACHE-TABLE--demo_offline: demo_offline [last modified: 2025-04-22 11:32:30] CACHE-TABLE--startpage: startpage [last modified: 2025-04-22 11:32:31] CACHE-TABLE--duckduckgo: duckduckgo [last modified: 2025-04-22 11:33:08] CACHE-TABLE--radio_browser: radio_browser [last modified: 2025-04-22 11:40:06] CACHE-TABLE--soundcloud: soundcloud [last modified: 2025-04-22 11:40:06] CACHE-TABLE--wolframalpha: wolframalpha These properties provide information about the state of the ExpireCache and control the behavior. For example, the maintenance intervals are controlled by the last modification date of the LAST_MAINTENANCE property and the hash value of the password can be used to detect whether the password has been changed (in this case the DB entries can no longer be decrypted and the entire cache must be discarded). Signed-off-by: Markus Heiser <markus.heiser@darmarit.de>
228 lines
6.8 KiB
Python
228 lines
6.8 KiB
Python
# SPDX-License-Identifier: AGPL-3.0-or-later
|
|
"""Search radio stations from RadioBrowser by `Advanced station search API`_.
|
|
|
|
.. _Advanced station search API:
|
|
https://de1.api.radio-browser.info/#Advanced_station_search
|
|
|
|
"""
|
|
from __future__ import annotations
|
|
|
|
import typing
|
|
import random
|
|
import socket
|
|
from urllib.parse import urlencode
|
|
import babel
|
|
from flask_babel import gettext
|
|
|
|
from searx.network import get
|
|
from searx.enginelib import EngineCache
|
|
from searx.enginelib.traits import EngineTraits
|
|
from searx.locales import language_tag
|
|
|
|
if typing.TYPE_CHECKING:
|
|
import logging
|
|
|
|
logger = logging.getLogger()
|
|
|
|
traits: EngineTraits
|
|
|
|
about = {
|
|
"website": 'https://www.radio-browser.info/',
|
|
"wikidata_id": 'Q111664849',
|
|
"official_api_documentation": 'https://de1.api.radio-browser.info/',
|
|
"use_official_api": True,
|
|
"require_api_key": False,
|
|
"results": 'JSON',
|
|
}
|
|
paging = True
|
|
categories = ['music', 'radio']
|
|
|
|
number_of_results = 10
|
|
|
|
station_filters = [] # ['countrycode', 'language']
|
|
"""A list of filters to be applied to the search of radio stations. By default
|
|
none filters are applied. Valid filters are:
|
|
|
|
``language``
|
|
Filter stations by selected language. For instance the ``de`` from ``:de-AU``
|
|
will be translated to `german` and used in the argument ``language=``.
|
|
|
|
``countrycode``
|
|
Filter stations by selected country. The 2-digit countrycode of the station
|
|
comes from the region the user selected. For instance ``:de-AU`` will filter
|
|
out all stations not in ``AU``.
|
|
|
|
.. note::
|
|
|
|
RadioBrowser has registered a lot of languages and countrycodes unknown to
|
|
:py:obj:`babel` and note that when searching for radio stations, users are
|
|
more likely to search by name than by region or language.
|
|
|
|
"""
|
|
|
|
CACHE: EngineCache
|
|
"""Persistent (SQLite) key/value cache that deletes its values after ``expire``
|
|
seconds."""
|
|
|
|
|
|
def init(_):
|
|
global CACHE # pylint: disable=global-statement
|
|
CACHE = EngineCache("radio_browser")
|
|
server_list()
|
|
|
|
|
|
def server_list() -> list[str]:
|
|
|
|
servers = CACHE.get("servers", [])
|
|
if servers:
|
|
return servers
|
|
|
|
# hint: can take up to 40sec!
|
|
ips = socket.getaddrinfo("all.api.radio-browser.info", 80, 0, 0, socket.IPPROTO_TCP)
|
|
for ip_tuple in ips:
|
|
_ip: str = ip_tuple[4][0] # type: ignore
|
|
url = socket.gethostbyaddr(_ip)[0]
|
|
srv = "https://" + url
|
|
if srv not in servers:
|
|
servers.append(srv)
|
|
|
|
# update server list once in 24h
|
|
CACHE.set(key="servers", value=servers, expire=60 * 60 * 24)
|
|
|
|
return servers
|
|
|
|
|
|
def request(query, params):
|
|
|
|
servers = server_list()
|
|
if not servers:
|
|
logger.error("Fetched server list is empty!")
|
|
params["url"] = None
|
|
return
|
|
|
|
server = random.choice(servers)
|
|
|
|
args = {
|
|
'name': query,
|
|
'order': 'votes',
|
|
'offset': (params['pageno'] - 1) * number_of_results,
|
|
'limit': number_of_results,
|
|
'hidebroken': 'true',
|
|
'reverse': 'true',
|
|
}
|
|
|
|
if 'language' in station_filters:
|
|
lang = traits.get_language(params['searxng_locale']) # type: ignore
|
|
if lang:
|
|
args['language'] = lang
|
|
|
|
if 'countrycode' in station_filters:
|
|
if len(params['searxng_locale'].split('-')) > 1:
|
|
countrycode = params['searxng_locale'].split('-')[-1].upper()
|
|
if countrycode in traits.custom['countrycodes']: # type: ignore
|
|
args['countrycode'] = countrycode
|
|
|
|
params['url'] = f"{server}/json/stations/search?{urlencode(args)}"
|
|
|
|
|
|
def response(resp):
|
|
results = []
|
|
|
|
json_resp = resp.json()
|
|
|
|
for result in json_resp:
|
|
url = result['homepage']
|
|
if not url:
|
|
url = result['url_resolved']
|
|
|
|
content = []
|
|
tags = ', '.join(result.get('tags', '').split(','))
|
|
if tags:
|
|
content.append(tags)
|
|
for x in ['state', 'country']:
|
|
v = result.get(x)
|
|
if v:
|
|
v = str(v).strip()
|
|
content.append(v)
|
|
|
|
metadata = []
|
|
codec = result.get('codec')
|
|
if codec and codec.lower() != 'unknown':
|
|
metadata.append(f'{codec} ' + gettext('radio'))
|
|
for x, y in [
|
|
(gettext('bitrate'), 'bitrate'),
|
|
(gettext('votes'), 'votes'),
|
|
(gettext('clicks'), 'clickcount'),
|
|
]:
|
|
v = result.get(y)
|
|
if v:
|
|
v = str(v).strip()
|
|
metadata.append(f"{x} {v}")
|
|
results.append(
|
|
{
|
|
'url': url,
|
|
'title': result['name'],
|
|
'thumbnail': result.get('favicon', '').replace("http://", "https://"),
|
|
'content': ' | '.join(content),
|
|
'metadata': ' | '.join(metadata),
|
|
'iframe_src': result['url_resolved'].replace("http://", "https://"),
|
|
}
|
|
)
|
|
|
|
return results
|
|
|
|
|
|
def fetch_traits(engine_traits: EngineTraits):
|
|
"""Fetch languages and countrycodes from RadioBrowser
|
|
|
|
- ``traits.languages``: `list of languages API`_
|
|
- ``traits.custom['countrycodes']``: `list of countries API`_
|
|
|
|
.. _list of countries API: https://de1.api.radio-browser.info/#List_of_countries
|
|
.. _list of languages API: https://de1.api.radio-browser.info/#List_of_languages
|
|
"""
|
|
# pylint: disable=import-outside-toplevel
|
|
|
|
init(None)
|
|
from babel.core import get_global
|
|
|
|
babel_reg_list = get_global("territory_languages").keys()
|
|
|
|
server = server_list()[0]
|
|
language_list = get(f'{server}/json/languages').json() # type: ignore
|
|
country_list = get(f'{server}/json/countries').json() # type: ignore
|
|
|
|
for lang in language_list:
|
|
|
|
babel_lang = lang.get('iso_639')
|
|
if not babel_lang:
|
|
# the language doesn't have any iso code, and hence can't be parsed
|
|
# print(f"ERROR: lang - no iso code in {lang}")
|
|
continue
|
|
try:
|
|
sxng_tag = language_tag(babel.Locale.parse(babel_lang, sep="-"))
|
|
except babel.UnknownLocaleError:
|
|
# print(f"ERROR: language tag {babel_lang} is unknown by babel")
|
|
continue
|
|
|
|
eng_tag = lang['name']
|
|
conflict = engine_traits.languages.get(sxng_tag)
|
|
if conflict:
|
|
if conflict != eng_tag:
|
|
print("CONFLICT: babel %s --> %s, %s" % (sxng_tag, conflict, eng_tag))
|
|
continue
|
|
engine_traits.languages[sxng_tag] = eng_tag
|
|
|
|
countrycodes = set()
|
|
for region in country_list:
|
|
# country_list contains duplicates that differ only in upper/lower case
|
|
_reg = region['iso_3166_1'].upper()
|
|
if _reg not in babel_reg_list:
|
|
print(f"ERROR: region tag {region['iso_3166_1']} is unknown by babel")
|
|
continue
|
|
countrycodes.add(_reg)
|
|
|
|
countrycodes = list(countrycodes)
|
|
countrycodes.sort()
|
|
engine_traits.custom['countrycodes'] = countrycodes
|