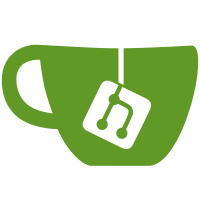
This patch brings two major changes: - ``Result.filter_urls(..)`` to pass a filter function for URL fields - The ``enabled_plugins:`` section in SearXNG's settings do no longer exists. To understand plugin development compile documentation: $ make docs.clean docs.live and read http://0.0.0.0:8000/dev/plugins/development.html There is no longer a distinction between built-in and external plugin, all plugins are registered via the settings in the ``plugins:`` section. In SearXNG, plugins can be registered via a fully qualified class name. A configuration (`PluginCfg`) can be transferred to the plugin, e.g. to activate it by default / *opt-in* or *opt-out* from user's point of view. built-in plugins ================ The built-in plugins are all located in the namespace `searx.plugins`. .. code:: yaml plugins: searx.plugins.calculator.SXNGPlugin: active: true searx.plugins.hash_plugin.SXNGPlugin: active: true searx.plugins.self_info.SXNGPlugin: active: true searx.plugins.tracker_url_remover.SXNGPlugin: active: true searx.plugins.unit_converter.SXNGPlugin: active: true searx.plugins.ahmia_filter.SXNGPlugin: active: true searx.plugins.hostnames.SXNGPlugin: active: true searx.plugins.oa_doi_rewrite.SXNGPlugin: active: false searx.plugins.tor_check.SXNGPlugin: active: false external plugins ================ SearXNG supports *external plugins* / there is no need to install one, SearXNG runs out of the box. - Only show green hosted results: https://github.com/return42/tgwf-searx-plugins/ To get a developer installation in a SearXNG developer environment: .. code:: sh $ git clone git@github.com:return42/tgwf-searx-plugins.git $ ./manage pyenv.cmd python -m \ pip install -e tgwf-searx-plugins To register the plugin in SearXNG add ``only_show_green_results.SXNGPlugin`` to the ``plugins:``: .. code:: yaml plugins: # ... only_show_green_results.SXNGPlugin: active: false Result.filter_urls(..) ====================== The ``Result.filter_urls(..)`` can be used to filter and/or modify URL fields. In the following example, the filter function ``my_url_filter``: .. code:: python def my_url_filter(result, field_name, url_src) -> bool | str: if "google" in url_src: return False # remove URL field from result if "facebook" in url_src: new_url = url_src.replace("facebook", "fb-dummy") return new_url # return modified URL return True # leave URL in field unchanged is applied to all URL fields in the :py:obj:`Plugin.on_result` hook: .. code:: python class MyUrlFilter(Plugin): ... def on_result(self, request, search, result) -> bool: result.filter_urls(my_url_filter) return True Signed-off-by: Markus Heiser <markus.heiser@darmarit.de>
94 lines
3.2 KiB
Python
94 lines
3.2 KiB
Python
# SPDX-License-Identifier: AGPL-3.0-or-later
|
|
# pylint: disable=missing-module-docstring,disable=missing-class-docstring,invalid-name
|
|
|
|
from parameterized.parameterized import parameterized
|
|
|
|
import searx.plugins
|
|
import searx.preferences
|
|
|
|
from searx.extended_types import sxng_request
|
|
from searx.result_types import Answer
|
|
|
|
from tests import SearxTestCase
|
|
from .test_utils import random_string
|
|
from .test_plugins import do_post_search
|
|
|
|
|
|
class PluginCalculator(SearxTestCase):
|
|
|
|
def setUp(self):
|
|
super().setUp()
|
|
engines = {}
|
|
|
|
self.storage = searx.plugins.PluginStorage()
|
|
self.storage.load_settings({"searx.plugins.calculator.SXNGPlugin": {"active": True}})
|
|
self.storage.init(self.app)
|
|
self.pref = searx.preferences.Preferences(["simple"], ["general"], engines, self.storage)
|
|
self.pref.parse_dict({"locale": "en"})
|
|
|
|
def test_plugin_store_init(self):
|
|
self.assertEqual(1, len(self.storage))
|
|
|
|
def test_pageno_1_2(self):
|
|
with self.app.test_request_context():
|
|
sxng_request.preferences = self.pref
|
|
query = "1+1"
|
|
answer = Answer(answer=f"{query} = {eval(query)}") # pylint: disable=eval-used
|
|
|
|
search = do_post_search(query, self.storage, pageno=1)
|
|
self.assertIn(answer, search.result_container.answers)
|
|
|
|
search = do_post_search(query, self.storage, pageno=2)
|
|
self.assertEqual(list(search.result_container.answers), [])
|
|
|
|
def test_long_query_ignored(self):
|
|
with self.app.test_request_context():
|
|
sxng_request.preferences = self.pref
|
|
query = f"1+1 {random_string(101)}"
|
|
search = do_post_search(query, self.storage)
|
|
self.assertEqual(list(search.result_container.answers), [])
|
|
|
|
@parameterized.expand(
|
|
[
|
|
("1+1", "2", "en"),
|
|
("1-1", "0", "en"),
|
|
("1*1", "1", "en"),
|
|
("1/1", "1", "en"),
|
|
("1**1", "1", "en"),
|
|
("1^1", "1", "en"),
|
|
("1,000.0+1,000.0", "2,000", "en"),
|
|
("1.0+1.0", "2", "en"),
|
|
("1.0-1.0", "0", "en"),
|
|
("1.0*1.0", "1", "en"),
|
|
("1.0/1.0", "1", "en"),
|
|
("1.0**1.0", "1", "en"),
|
|
("1.0^1.0", "1", "en"),
|
|
("1.000,0+1.000,0", "2.000", "de"),
|
|
("1,0+1,0", "2", "de"),
|
|
("1,0-1,0", "0", "de"),
|
|
("1,0*1,0", "1", "de"),
|
|
("1,0/1,0", "1", "de"),
|
|
("1,0**1,0", "1", "de"),
|
|
("1,0^1,0", "1", "de"),
|
|
]
|
|
)
|
|
def test_localized_query(self, query: str, res: str, lang: str):
|
|
with self.app.test_request_context():
|
|
self.pref.parse_dict({"locale": lang})
|
|
sxng_request.preferences = self.pref
|
|
answer = Answer(answer=f"{query} = {res}")
|
|
|
|
search = do_post_search(query, self.storage)
|
|
self.assertIn(answer, search.result_container.answers)
|
|
|
|
@parameterized.expand(
|
|
[
|
|
"1/0",
|
|
]
|
|
)
|
|
def test_invalid_operations(self, query):
|
|
with self.app.test_request_context():
|
|
sxng_request.preferences = self.pref
|
|
search = do_post_search(query, self.storage)
|
|
self.assertEqual(list(search.result_container.answers), [])
|