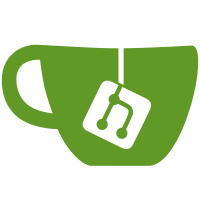
The class ReslutContainer has been revised, it can now handle the typed Result items of classes: - MainResult - LegacyResult (a dict wrapper for backward compatibility) Due to the now complete typing of theses three clases, instead of the *getitem* accesses, the fields can now be accessed directly via attributes (which is also supported by the IDE). Signed-off-by: Markus Heiser <markus.heiser@darmarit.de>
61 lines
2.2 KiB
Python
61 lines
2.2 KiB
Python
# SPDX-License-Identifier: AGPL-3.0-or-later
|
|
# pylint: disable=missing-module-docstring,disable=missing-class-docstring,invalid-name
|
|
|
|
|
|
from searx.result_types import LegacyResult
|
|
from searx.results import ResultContainer
|
|
from tests import SearxTestCase
|
|
|
|
|
|
class ResultContainerTestCase(SearxTestCase):
|
|
# pylint: disable=use-dict-literal
|
|
|
|
TEST_SETTINGS = "test_result_container.yml"
|
|
|
|
def test_empty(self):
|
|
container = ResultContainer()
|
|
self.assertEqual(container.get_ordered_results(), [])
|
|
|
|
def test_one_result(self):
|
|
result = dict(url="https://example.org", title="title ..", content="Lorem ..")
|
|
|
|
container = ResultContainer()
|
|
container.extend("google", [result])
|
|
container.close()
|
|
|
|
self.assertEqual(len(container.get_ordered_results()), 1)
|
|
|
|
res = LegacyResult(result)
|
|
res.normalize_result_fields()
|
|
self.assertIn(res, container.get_ordered_results())
|
|
|
|
def test_one_suggestion(self):
|
|
result = dict(suggestion="lorem ipsum ..")
|
|
|
|
container = ResultContainer()
|
|
container.extend("duckduckgo", [result])
|
|
container.close()
|
|
|
|
self.assertEqual(len(container.get_ordered_results()), 0)
|
|
self.assertEqual(len(container.suggestions), 1)
|
|
self.assertIn(result["suggestion"], container.suggestions)
|
|
|
|
def test_merge_url_result(self):
|
|
# from the merge of eng1 and eng2 we expect this result
|
|
result = LegacyResult(
|
|
url="https://example.org", title="very long title, lorem ipsum", content="Lorem ipsum dolor sit amet .."
|
|
)
|
|
result.normalize_result_fields()
|
|
eng1 = dict(url=result.url, title="short title", content=result.content, engine="google")
|
|
eng2 = dict(url="http://example.org", title=result.title, content="lorem ipsum", engine="duckduckgo")
|
|
|
|
container = ResultContainer()
|
|
container.extend(None, [eng1, eng2])
|
|
container.close()
|
|
|
|
result_list = container.get_ordered_results()
|
|
self.assertEqual(len(container.get_ordered_results()), 1)
|
|
self.assertIn(result, result_list)
|
|
self.assertEqual(result_list[0].title, result.title)
|
|
self.assertEqual(result_list[0].content, result.content)
|