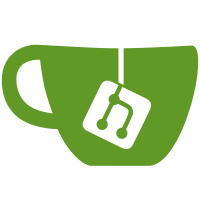
A local development server can be launched by one of these command lines:: $ flask --app searx.webapp run $ python -m searx.webapp The different ways of starting the server should lead to the same result, which is generally the case. However, if the modules are reloaded after code changes (reload option), it must be avoided that the application is initialized twice at startup. We have already discussed this in 2022 [1][2]. Further information on this topic can be found in [3][4][5]. To test a bash in the ./local environment was started and the follwing commands had been executed:: $ ./manage pyenv.cmd bash --norc --noprofile (py3) SEARXNG_DEBUG=1 flask --app searx.webapp run --reload (py3) SEARXNG_DEBUG=1 python -m searx.webapp Since the generic parts of the docs also initialize the app to generate doc from it, the build of the docs was also tested:: $ make docs.clean docs.live [1] https://github.com/searxng/searxng/pull/1656#issuecomment-1214198941 [2] https://github.com/searxng/searxng/pull/1616#issuecomment-1206137468 [3] https://flask.palletsprojects.com/en/stable/api/#flask.Flask.run [4] https://github.com/pallets/flask/issues/5307#issuecomment-1774646119 [5] https://stackoverflow.com/a/25504196 Signed-off-by: Markus Heiser <markus.heiser@darmarit.de>
93 lines
2.6 KiB
Python
93 lines
2.6 KiB
Python
# SPDX-License-Identifier: AGPL-3.0-or-later
|
|
# pylint: disable=missing-module-docstring,disable=missing-class-docstring,invalid-name
|
|
|
|
import pathlib
|
|
import os
|
|
import aiounittest
|
|
|
|
|
|
os.environ.pop('SEARXNG_SETTINGS_PATH', None)
|
|
os.environ['SEARXNG_DISABLE_ETC_SETTINGS'] = '1'
|
|
|
|
|
|
class SearxTestLayer:
|
|
"""Base layer for non-robot tests."""
|
|
|
|
__name__ = 'SearxTestLayer'
|
|
|
|
@classmethod
|
|
def setUp(cls):
|
|
pass
|
|
|
|
@classmethod
|
|
def tearDown(cls):
|
|
pass
|
|
|
|
@classmethod
|
|
def testSetUp(cls):
|
|
pass
|
|
|
|
@classmethod
|
|
def testTearDown(cls):
|
|
pass
|
|
|
|
|
|
class SearxTestCase(aiounittest.AsyncTestCase):
|
|
"""Base test case for non-robot tests."""
|
|
|
|
layer = SearxTestLayer
|
|
|
|
SETTINGS_FOLDER = pathlib.Path(__file__).parent / "unit" / "settings"
|
|
TEST_SETTINGS = "test_settings.yml"
|
|
|
|
def setUp(self):
|
|
self.init_test_settings()
|
|
|
|
def setattr4test(self, obj, attr, value):
|
|
"""setattr(obj, attr, value) but reset to the previous value in the
|
|
cleanup."""
|
|
previous_value = getattr(obj, attr)
|
|
|
|
def cleanup_patch():
|
|
setattr(obj, attr, previous_value)
|
|
|
|
self.addCleanup(cleanup_patch)
|
|
setattr(obj, attr, value)
|
|
|
|
def init_test_settings(self):
|
|
"""Sets ``SEARXNG_SETTINGS_PATH`` environment variable an initialize
|
|
global ``settings`` variable and the ``logger`` from a test config in
|
|
:origin:`tests/unit/settings/`.
|
|
"""
|
|
|
|
os.environ['SEARXNG_SETTINGS_PATH'] = str(self.SETTINGS_FOLDER / self.TEST_SETTINGS)
|
|
|
|
# pylint: disable=import-outside-toplevel
|
|
import searx
|
|
import searx.locales
|
|
import searx.plugins
|
|
import searx.search
|
|
import searx.webapp
|
|
|
|
# https://flask.palletsprojects.com/en/stable/config/#builtin-configuration-values
|
|
# searx.webapp.app.config["DEBUG"] = True
|
|
searx.webapp.app.config["TESTING"] = True # to get better error messages
|
|
searx.webapp.app.config["EXPLAIN_TEMPLATE_LOADING"] = True
|
|
|
|
searx.init_settings()
|
|
searx.plugins.initialize(searx.webapp.app)
|
|
|
|
# searx.search.initialize will:
|
|
# - load the engines and
|
|
# - initialize searx.network, searx.metrics, searx.processors and searx.search.checker
|
|
|
|
searx.search.initialize(
|
|
enable_checker=True,
|
|
check_network=True,
|
|
enable_metrics=searx.get_setting("general.enable_metrics"), # type: ignore
|
|
)
|
|
|
|
# pylint: disable=attribute-defined-outside-init
|
|
self.app = searx.webapp.app
|
|
self.client = searx.webapp.app.test_client()
|