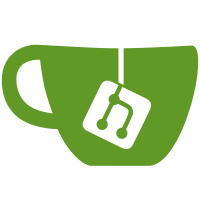
Comparing changes: https://github.com/smarty-php/smarty/compare/v4.3.1...v4.4.1 It is noticeable that Smarty 4.3.1 does not officially support PHP 8.3. Is only supported with 4.4.0. Remark: During tests with Smarty 4.5.1, it was noticed that the following warning occurs: Deprecated: Using the unregistered function "function_exists" in a template is deprecated and will be removed in a future version. Use Smarty::registerPlugin to explicitly register a custom modifier. As of Smarty 5.X.X, templates must be revised again. The Smarty release 5.0.2 is already officially available. However, integration into FlatPress is not entirely trivial.
79 lines
2.3 KiB
PHP
79 lines
2.3 KiB
PHP
<?php
|
|
/**
|
|
* Smarty Internal Plugin Resource Stream
|
|
* Implements the streams as resource for Smarty template
|
|
*
|
|
* @package Smarty
|
|
* @subpackage TemplateResources
|
|
* @author Uwe Tews
|
|
* @author Rodney Rehm
|
|
*/
|
|
|
|
/**
|
|
* Smarty Internal Plugin Resource Stream
|
|
* Implements the streams as resource for Smarty template
|
|
*
|
|
* @link https://php.net/streams
|
|
* @package Smarty
|
|
* @subpackage TemplateResources
|
|
*/
|
|
class Smarty_Internal_Resource_Stream extends Smarty_Resource_Recompiled
|
|
{
|
|
/**
|
|
* populate Source Object with meta data from Resource
|
|
*
|
|
* @param Smarty_Template_Source $source source object
|
|
* @param Smarty_Internal_Template $_template template object
|
|
*
|
|
* @return void
|
|
*/
|
|
public function populate(Smarty_Template_Source $source, Smarty_Internal_Template $_template = null)
|
|
{
|
|
if (strpos($source->resource, '://') !== false) {
|
|
$source->filepath = $source->resource;
|
|
} else {
|
|
$source->filepath = str_replace(':', '://', $source->resource);
|
|
}
|
|
$source->uid = false;
|
|
$source->content = $this->getContent($source);
|
|
$source->timestamp = $source->exists = !!$source->content;
|
|
}
|
|
|
|
/**
|
|
* Load template's source from stream into current template object
|
|
*
|
|
* @param Smarty_Template_Source $source source object
|
|
*
|
|
* @return string template source
|
|
*/
|
|
public function getContent(Smarty_Template_Source $source)
|
|
{
|
|
$t = '';
|
|
// the availability of the stream has already been checked in Smarty_Resource::fetch()
|
|
$fp = fopen($source->filepath, 'r+');
|
|
if ($fp) {
|
|
while (!feof($fp) && ($current_line = fgets($fp)) !== false) {
|
|
$t .= $current_line;
|
|
}
|
|
fclose($fp);
|
|
return $t;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* modify resource_name according to resource handlers specifications
|
|
*
|
|
* @param Smarty $smarty Smarty instance
|
|
* @param string $resource_name resource_name to make unique
|
|
* @param boolean $isConfig flag for config resource
|
|
*
|
|
* @return string unique resource name
|
|
*/
|
|
public function buildUniqueResourceName(Smarty $smarty, $resource_name, $isConfig = false)
|
|
{
|
|
return get_class($this) . '#' . $resource_name;
|
|
}
|
|
}
|