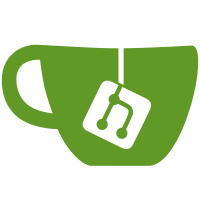
Comparing changes: https://github.com/smarty-php/smarty/compare/v4.3.1...v4.4.1 It is noticeable that Smarty 4.3.1 does not officially support PHP 8.3. Is only supported with 4.4.0. Remark: During tests with Smarty 4.5.1, it was noticed that the following warning occurs: Deprecated: Using the unregistered function "function_exists" in a template is deprecated and will be removed in a future version. Use Smarty::registerPlugin to explicitly register a custom modifier. As of Smarty 5.X.X, templates must be revised again. The Smarty release 5.0.2 is already officially available. However, integration into FlatPress is not entirely trivial.
74 lines
1.7 KiB
PHP
74 lines
1.7 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Smarty compiler exception class
|
|
*
|
|
* @package Smarty
|
|
*/
|
|
class SmartyCompilerException extends SmartyException
|
|
{
|
|
/**
|
|
* The constructor of the exception
|
|
*
|
|
* @param string $message The Exception message to throw.
|
|
* @param int $code The Exception code.
|
|
* @param string|null $filename The filename where the exception is thrown.
|
|
* @param int|null $line The line number where the exception is thrown.
|
|
* @param Throwable|null $previous The previous exception used for the exception chaining.
|
|
*/
|
|
public function __construct(
|
|
string $message = "",
|
|
int $code = 0,
|
|
?string $filename = null,
|
|
?int $line = null,
|
|
Throwable $previous = null
|
|
) {
|
|
parent::__construct($message, $code, $previous);
|
|
|
|
// These are optional parameters, should be be overridden only when present!
|
|
if ($filename) {
|
|
$this->file = $filename;
|
|
}
|
|
if ($line) {
|
|
$this->line = $line;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function __toString()
|
|
{
|
|
return ' --> Smarty Compiler: ' . $this->message . ' <-- ';
|
|
}
|
|
|
|
/**
|
|
* @param int $line
|
|
*/
|
|
public function setLine($line)
|
|
{
|
|
$this->line = $line;
|
|
}
|
|
|
|
/**
|
|
* The template source snippet relating to the error
|
|
*
|
|
* @type string|null
|
|
*/
|
|
public $source = null;
|
|
|
|
/**
|
|
* The raw text of the error message
|
|
*
|
|
* @type string|null
|
|
*/
|
|
public $desc = null;
|
|
|
|
/**
|
|
* The resource identifier or template name
|
|
*
|
|
* @type string|null
|
|
*/
|
|
public $template = null;
|
|
}
|